Arrays.sort() 를 사용하게 되면 오름차순으로 정렬이 된다.
2차원 배열이나, 객체 배열을 정렬하고 싶을때는
Arrays.sort()를 확장할 수 있어야한다.
우선 Arrays.sort의 기본형을 알아보자
Arrays.sort()
아래 배열이 있다.
int arr[] = {1,4,2,3,7};
1.오름차순 정렬
Arrays.sort(arr);
System.out.println(Arrays.toString(arr));
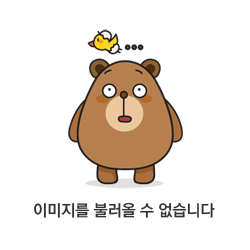
2.내림차순 정렬
:배열 타입을 기본형이 아닌 참조형으로 변경해야 한다.
<참고>
기본 타입(primitive type) : 정수형, 실수형, 문자형 그리고 논리형 타입
타입(data type)은 해당 데이터가 메모리에 어떻게 저장되고, 프로그램에서 어떻게 처리되어야 하는지를 명시적으로 알려주는 역할을 합니다.
자바에서는 여러 형태의 타입을 미리 정의하여 제공하고 있는데, 이것을 기본 타입(primitive type)이라고 합니다.
자바의 기본 타입은 모두 8종류가 제공되며, 크게는 정수형, 실수형, 문자형 그리고 논리형 타입으로 나눌 수 있습니다.
import java.util.Collections;
Integer arr[] = {1,4,2,3,7}; =>배열을 참조형으로 변경해야한다.
Arrays.sort(arr, Collections.reverseOrder());
이제는 객체 개념으로 넘어가서 예를 들어 Student라는 클래스를 생성을 했다.Student는 나이와 이름이라는 속성(프로퍼티)을 가지고 있다.
public class Student {
int age;
String name;
public Student(int age,String name) {
this.age = age;
this.name = name;
}
}
20명의 학생이 있는데 학생들을 나이별로 정렬을 하고싶다.
이때에는 Comparator을 이용하여 정렬을 할 수 있다.
Arrays.sort() => Comparator 사용하기
1.Comparator 클래스 만들기
:인터페이스를 implements하는 클래스를 만들어서 기준 정하기
-MyCompare 클래스
/*MyCompare 클래스*/
class MyCompare implements Comparator<Student>{
@override
public int compare(Student s1,Student s2){
return s1.age-s2.age;
}
}
-Main 클래스
public class Main {
int length = 5;
int studentNum = 0;
//객체배열 생성
Student students[] = new Student[length];
//학생 5명 생성
students[0] = new Student(5,++studentNum);
students[1] = new Student(10,++studentNum);
students[2] = new Student(7,++studentNum);
students[3] = new Student(6,++studentNum);
students[4] = new Student(8,++studentNum);
MyCompare compares = new MyCompare();
Arrays.sort(students,compares);
}
2.Comparator 클래스 만들기
:객체 선언 및 오버라이딩
Comparator <Student> myCompare = new Comparator<Student>(){
@override
public int compare(Student s1,Student s2) {
return s1.age-s2.age;
}
};
Arrays.sort(students,myCompare);
3.익명 객체 방식
:변수 없이 익명객체 방식
Arrays.sort(myArr,new Comparator<Student>(){
public int compare(Student s1,Student s2){
return s1.age-s2.age
}
});
4.람다식
Arrays.sort(students,(s1,s2)->{
return s1.age-s2.age;
});
+추가 이차원배열 풀기
import java.util.Arrays;
import java.util.Scanner;
public class Q11650_2 {
/*
2차원 평면 위의 점 N개가 주어진다. 좌표를 x좌표가 증가하는 순으로, x좌표가 같으면 y좌표가 증가하는 순서로 정렬한 다음 출력하는 프로그램을 작성하시오.
*/
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
int N = 0;
// 횟수
N = scan.nextInt();
int point[][] = new int[N][2];
// int point[][] = {{1, 2}, {1, 5}, {6, 7}, {3, 4}, {2, 1}};
// 입력 부분
for(int i = 0; i < N ; i++) {
point[i][0]= scan.nextInt(); // X좌표
point[i][1]= scan.nextInt(); // X좌표
}
// 정렬
Arrays.sort(point, (p1,p2)->{
if(p1[0] == p2[0]) { // X좌표가 동일시
return p1[1]-p2[1];
}
return p1[0]-p2[0];
});
// 출력 부분
for(int j = 0; j < N ; j++) {
System.out.println(point[j][0]+" "+point[j][1]);
}
}
}
'한국폴리텍대학교 수업정리 > 자바' 카테고리의 다른 글
[2024-03-05]JAVA SE,JRE,JDK,메이저,마이너,LTS,명시적 형변환,묵시적형변환 (0) | 2024.03.05 |
---|
- Total
- Today
- Yesterday
- data속성일때 선택자
- 네이버 노출
- 캡처링
- RSS태그#지식
- CheckBox
- EQ
- 도메인이해
- react
- 네이버노출
- 다짐#개발자#Vlog
- CSP#콘텐츠보안정책#style코드 작성시
- onClick
- 도메인개념
- p
- domain
- jQuey
- 검색결과
- 네이버
- Develop 사전
- 배열선택자
- ㅏ
- 유입증가시키는방법
- 선택자
- PP
- js
- ㅂㅜ티
- 티스토리
- 버블링
- ~`
- 데이터베이스#RDBMS#스키마
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |